reCAPTCHA with Ember
Ever wanted to add a reCAPTCHA to your Ember app to prevent automated software (robots 🤖) from generating requests? Good news! There’s already an add-on that has you covered. It’s really easy to use too, which is great. I recently added this to one of our signup forms.
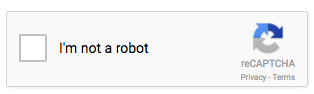
Head on over to the Google reCAPTCHA site and click the “Get reCAPTCHA” button. If you’re already logged in with a Google account, hooray! If not, you’ll need a Google account to use this service. Once you get logged in, it’s really easy. I won’t go through all of the steps to get all setup, but once you let them know your domains and other settings, you should have two keys. A “site key” and a “secret key”. The site key is used in your HTML for client-side verification. These are public. The secret key is used for communication between your site and Google. As they suggest, keep it a secret.
Install the add on
From your Ember app, run the below cli command:
ember install ember-g-recaptcha Setup your site key and add the component You have two ways of specifying your site key in your Ember app. One option is to set it on your ENV variable as the read me suggests:
var ENV = {
// ...
gReCaptcha: {
siteKey: 'your-recaptcha-site-key',
},
// ...
}
Another option is to set it on the component directly.
{{g-recaptcha size="normal" sitekey='your-recaptcha-site-key' onSuccess=(action "onCaptchaResolved")}}
Note: If you have automation tests running in test environments or don’t want to create a site key just yet, you can use the one provided by Google’s FAQ page. This test site key doesn’t require you/your tests to prove you’re not a robot. Just click the checkbox and be on your way. Note the nice little message about it being a test-only key.
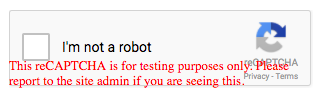
Content Security Policy
By default, Ember specifies a content security policy. Update your settings as suggested by the Google FAQS. It should look something like:
var ENV = {
//
contentSecurityPolicy: {
//
'style-src': "'self' 'unsafe-inline'",
'script-src':
"'self' https://www.google.com/recaptcha/ https://www.gstatic.com/recaptcha/",
'frame-src': "'self' https://www.google.com/recaptcha/",
},
}
onSuccess Action
You’ll want to use a closure action for when the user passes the reCAPTCHA. This can be done by setting it up in your template and then adding the action in your actions hash:
// component.hbs
{{g-recaptcha size="normal" sitekey='your-recaptcha-site-key' onSuccess=(action "onCaptchaResolved")}}
// component.js
actions: {
onCaptchaResolved(reCaptchaResponse) {
// Validate the reCAPTCHA response, maybe do something in your component to allow the user to proceed, etc.
},
}
Validate the reCAPTCHA response
The server request you need to make to validate the reCAPTCHA response is:
// --> POST
https://www.google.com/recaptcha/api/siteverify?secret=<SECRET_KEY_FROM_ADMIN_CONSOLE>&response=<VALUE_FROM_onCaptchaResolved>
The response from the POST currently looks something this:
{
"success": true,
"challenge_ts": "2017-04-21T14:24:37Z",
"hostname": "localhost"
}
And that’s the waaaaaaaaay the news goes Hope this was somewhat helpful in showing how easy it is to add a reCAPTCHA to your Ember app. Have fun!