Setting up ESLint in Ember
Looking to setup a linter for your Ember app? I’ve setup ESLint with a handful of repos lately, so I thought it was time for another post on how to get up and running quickly. I’m going to assume you already know about ESLint and linting in general. I’ll try and keep it short and sweet.
(Note: Looks like a new ESLint plugin for Ember best practices just surfaced. Take a look at the README, but continue to read along for quick setup!)
Step #1: Install ember-cli-eslint
Note: For Ember 2.12 and higher, ember-cli will do this step for you!
Go into your Ember app directory and run:
ember install ember-cli-eslint After running the ember install above, you’ll see a message like below. By default, Ember apps create those JSHint config files for you. You may want to double check what’s in those files, but if you haven’t touched them at all, it’s more than likely safe to whack ‘em.
No matter what option you choose, you’ll find a .eslintrc.js file in the root of your directory. This file is where you will specify linting rules. Yay!
Step #2a: Create a test rule
If you want to do a quick test to see if things are working, modify your .eslintrc.js file to look something like this:
module.exports = {
// other stuff
rules: {
'no-console': 'error', // error if console statements
},
}
This is one of the many rules provided by ESLint, where it will error if there are any console statements. Easy enough.
Step #2b: Make sure we get a linting error
What’s really nice is that the linting rules are evaluated when running ember t. Just go into one of your JS files, add a console.log('hi'); statement and run ember t. You should get a linting error that looks like:
ember-quickstart/app/components/pasta-selector.js
6:13 error Unexpected console statement no-console
✖ 1 problem (1 error, 0 warnings)
Sweet — things appear to be working!
Step #3: Add real rules
Depending on if you’re working solo or on a team, you may want to follow certain rules. The available rules can be found on the ESLint website. Just add them like we added our test rule above. Your JS files are now linted — good job!
Step #4: Install ember-cli-template-lint
So we are linting JS files now, what about our hbs templates? Can we lint those? Of course!
ember install ember-cli-template-lint
After running the above command, a .template-lintrc.js file is created. Guess what goes in there? Template rules!
Step #5a: Create a test template rule
We’ll do a quick test, just to ensure everything is setup for linting our templates. Add the following to your lintrc file:
module.exports = {
extends: 'recommended',
rules: {
'bare-strings': true, // don't allow bare strings
},
}
Step #5b: Make sure we get a linting error
Go over to one of your hbs files and add a bare string like:
<div>Should have template error</div>
Then just run the trusty ember t command, and you should see:
bare-strings: Non-translated string used (ember-quickstart/templates/components/pasta-selector @ L1:C5):
`Should have template error`
===== 1 Template Linting Error
These errors show up at the top of the console output, so if you’re working with a big code base with a lot of errors, you may miss them. Luckily though, you can either a) scroll up or b) notice they failed as tests via the message:
not ok 4 PhantomJS 2.1 - TemplateLint - ember-quickstart/templates/components/pasta-selector.hbs: should pass TemplateLint
Step #6: Add real template lint rules
The available templates rules can be found on the ember-template-lint README. Here’s a brief example:
module.exports = {
extends: 'recommended',
rules: {
'bare-strings': true,
'triple-curlies': false,
'block-indentation': 4,
},
}
Step #7: Setup your editor
If you use VSCode, this ESLint extension is awesome. My favorite part is that it just works out of the box after installing. No other setup was required (at least for me…). The extension also has an auto-fix command, where it will automatically resolve any lint errors. This is done via the lightbulb in the gutter or via the ESLint: Fix all auto-fixable problems command.
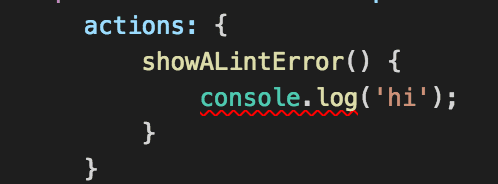
Other editors have similar plugins/extensions, so just google away for your answers. It’s nice to be working in a file and see any lint errors right away, before even running ember t.
That’s all
Adding ESLint to your Ember project isn’t that difficult, which is really nice. If you have any issues, the bulk of them will likely be editor extension/plugin related. If you try this out and hit some snags, let me know and I’d be glad to help! Happy linting!